Meteor preload Data, kind of SSR, Svelte5
neat trick to make your 1st load fast and not wait on a Meteor Subscription or a Meteor Method is to render it into the window scope as a variable.
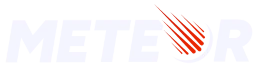
import { Meteor } from "meteor/meteor";
import { WebApp } from "meteor/webapp";
import { onPageLoad } from "meteor/server-render";
import { SearchCollection } from "/imports/api/search/collection.js";
const dataCache = new Map();
WebApp.handlers.use("/search/:id", async (req, res, next) => {
console.log("search/:id", req.params.id);
const id = req.params.id;
if (!dataCache.has(req.baseUrl)) {
const search = await SearchCollection.findOneAsync({ _id: id });
dataCache.set(req.baseUrl, search);
}
next();
});
const normalizePath = (path) => path.replace(/\/+$/, "");
onPageLoad(async (sink) => {
const path = normalizePath(sink.request.url.pathname);
const data = dataCache.get(path);
if (data) {
sink.appendToHead(`<script>window.__DATA__ = ${JSON.stringify(data)}</script>`);
}
});
and in your view:
<script>
import { onMount, onDestroy } from "svelte";
let { searchId } = $props();
let search = $state(null);
let sub, computation;
$effect(() => {
// Clean up previous subscription and computation
sub?.stop();
computation?.stop();
if (searchId) {
sub = Meteor.subscribe("searchResults.updates", searchId);
computation = Tracker.autorun(() => {
if (sub.ready()) {
search = SearchCollection.findOne({ _id: searchId });
}
});
}
return () => {
sub?.stop();
computation?.stop();
};
});
onMount(() => {
// console.log("data", window.__DATA__);
if (window.__DATA__) {
search = window.__DATA__;
}
});
</script>
Woud be cool to take this further and actually render templates server side completely, instead of just passing the data.
Links:
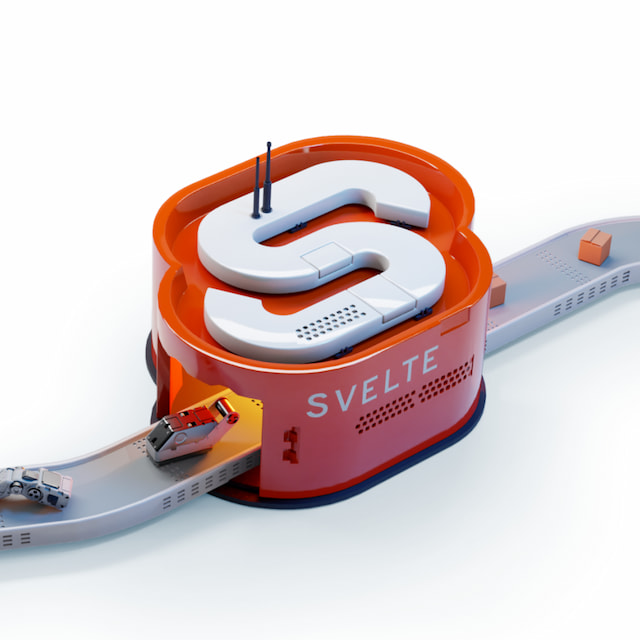